The following code takes the raw input from Fresh Water Tank No.1 and converts it to liters based on the calibration data. It is a simple function to find the upper and lower raw values and then use the same ratio between them to determine the water level. The first and last records of the calibration data are there in case the raw data is outside the previously measured range, and will show a full or empty tank respectively.
let rawFreshTank1Level = msg.payload;
const freshTank1Calibration = [
[10000, 132.344827586207],
[3359, 132.344827586207],
[3284, 128.48275862069],
[3242, 126.96551724138],
[3128, 121.034482758621],
[3051, 115.103448275862],
[3006, 109.448275862069],
[2948, 103.586206896552],
[2880, 97.7241379310347],
[2787, 91.7241379310347],
[2635, 85.7241379310347],
[2546, 79.7241379310347],
[2447, 73.7241379310347],
[1974, 68.0000000000002],
[1805, 62.068965517242],
[1649, 56.137931034483],
[1485, 50.137931034483],
[1292, 44.137931034483],
[1120, 38.068965517242],
[913, 32],
[658, 25.862068965517],
[605, 19.793103448276],
[535, 13.655172413793],
[527, 7.379310344828],
[519, 0]]
const freshTank1dimensions = [freshTank1Calibration.length, freshTank1Calibration[0].length];
let scaledFreshTank1Level = 0;
for (let i = 0; i < freshTank1dimensions[0]-1; i++) {
if (rawFreshTank1Level <= freshTank1Calibration[i][0] && rawFreshTank1Level >= freshTank1Calibration[i+1][0]) {
scaledFreshTank1Level = Math.round((rawFreshTank1Level - freshTank1Calibration[i + 1][0]) / (freshTank1Calibration[i][0] - freshTank1Calibration[i + 1][0]) * (freshTank1Calibration[i][1] - freshTank1Calibration[i + 1][1]) + freshTank1Calibration[i + 1][1]);
break;
}
}
msg.payload = scaledFreshTank1Level;
return msg;
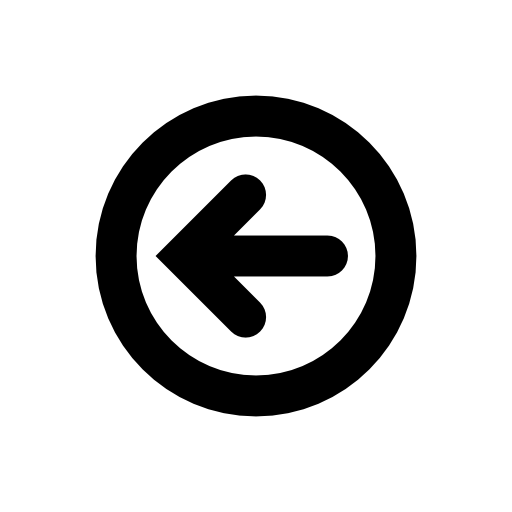